On the Silverlight forum I've seen a lot of questions regarding authentication and security.
I figured I'd write something down from my perspective as an ASP.NET developer. This isn't the only way to do it and probably not the best, but it does what I need it to do and it might get you started in the right direction.
IMPORTANT NOTE: This article is built with the assumption that all files requested are handled by ASP.NET and not by IIS (so let .net dll handle the wildcard extension *).
Click here to download the source
1. How and why
ASP.NET provides excellent methods for securing web applications using WindowsAuthentication or FormsAuthentication. And creating your own MembershipProvider allows you to authenticate using any type of database or other data storage.For this example I chose to use FormsAuthentication because this gives you lots of freedom and flexibility to expand or adjust in a later stage of the build of your project.
2. Securing the ASP.NET application
In this example we will secure the application and will test it using 2 types of request:- a file which is in a secured folder on the server
- a WCF webservice secured method
I used a standard Silverlight Application Project template in Visual Studio with a Web Application project, not a Website. This will probably also work with the Website, but to prevent problems better use the WebApplication type.
2.1 Changes to the Web.Config
First of all we're going to configure the ASP.NET Web Application to use FormsAuthentication to secure a folder.Create a folder named "Secure" in the root of the ASP.NET Web Application (website from here on).
Open the Web.Config and locate the following section:
Change it to this:
Second thing to do in the Web.Config is to add the following section just after the tag:
That's it. We're done securing the folder Secure in our website. To verify this, create an XML file in the Secure folder with the following content:
You can only see this if you're logged in.
Now run your website pressing F5 and try to open the XML file. If everything went as planned you should see the application redirected you to a login.aspx which doesn't exist. We won't create one in this tutorial because we'll be using Silverlight to do the logging in and not ASP.NET.
Next thing we're going to do is to create the WCF webservice that we'll use for logging in the website.
2.2 Create a WCF Authentication Service
We'll be using a standard Silverlight WCF Service for this. I always like to keep my application tidy so I created a folder in the root of my website named "WebServices".
In that folder, create a "Silverlight-enabled WCF Service" which you can find in the dialog that appears when you click on Add => New Item and when you select the Silverlight Category. Name the service "AuthenticationService".
In the created WCF service there is a method already created for you:
[OperationContract]
public void DoWork()
{
// Add your operation implementation here
return;
}
Replace this method with the following code:
[OperationContract]
public bool Authenticate(string Username, string Password)
{
if (FormsAuthentication.Authenticate(Username, Password))
{
FormsAuthentication.SetAuthCookie(Username, false);
return true;
}
return false;
}
As you can see is that all we did was create a method with a username and password parameter which uses the two default FormsAuthentication methods, Authenticate and SetAuthCookie, to enable security. The reason we need the SetAuthCookie is because it fills the
HttpContext.Current.Userobject which we can read out later on to verify if a user is logged in and which user it is.
That covers the authentication part for now. Next, we'll create the Silverlight Application with the login form.
2.3 Creating the login form in Silverlight
Open the Page.xaml in Expression Blend. In the LayoutRoot, add three Grids:
- LoginForm
- ResultForm
- TestForm
Make sure the ResultForm is positioned at about the same position as the LoginForm and the the visibility of the ResultForm is set to Collapsed.
On the LoginForm, add the textblocks (labels), textboxes and a button needed to create login form so that it looks like this:
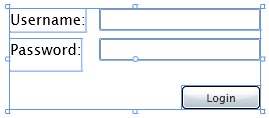
On the ResultForm, add a textblox with a default text of:
Login succeeded. You can continue with the application
On the TestForm, add 2 buttons and a large Textblock:
- ButtonGetMyBalance
- ButtonGetXmlFile
- TextBlockResult
When you're done you should have something similar to this:
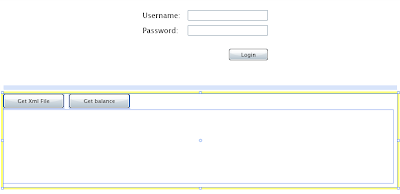
Now we're done with preparing the interface and we'll proceed to the coding of the application. Open the Page.xaml.cs with Visual Studio 2008.
2.4 Calling the AuthenticationService
Before calling the service, we need to add the reference to the service to the Silverlight Application.
Right-click on the Service Reference folder in the Silverlight Application project, and select "Add Service Reference".
Next, click on the "Discover" button that is in the pop-up window that will appear.
After the discovery is completed, you'll see the AuthenticationService appear in the box "Services".
Select it and change the "Namespace" to: "AuthenticationService". Now press OK and the service will be added to you project.
Now, let's continue in the Page.xaml.cs.
In the Page() constructor, add the following lines of code after the InitializeComponent() method:
ButtonLogin.Click += new RoutedEventHandler(ButtonLogin_Click);
ButtonGetXmlFile.Click += new RoutedEventHandler(ButtonGetXmlFile_Click);
Next, add the code for getting the Xml file using a WebClient:
void ButtonGetXmlFile_Click(object sender, RoutedEventArgs e)
{
WebClient webclient = new WebClient();
webclient.DownloadStringCompleted += new DownloadStringCompletedEventHandler(webclient_DownloadStringCompleted);
webclient.DownloadStringAsync(new Uri("../Secure/TestFile.xml", UriKind.Relative));
}
void webclient_DownloadStringCompleted(object sender, DownloadStringCompletedEventArgs e)
{
if (e.Error == null && !string.IsNullOrEmpty(e.Result))
{
TextBlockResult.Text = e.Result;
}
else
{
if (e.Error != null)
TextBlockResult.Text = e.Error.Message;
else
TextBlockResult.Text = "Please login first";
}
}
Now create the event handler for the ButtonLogin.Click event:
private void ButtonLogin_Click(object sender, RoutedEventArgs e)
{
AuthenticationService.AuthenticationServiceClient authService = new AuthenticationService.AuthenticationServiceClient();
authService.AuthenticateCompleted += new EventHandler(authService_AuthenticateCompleted);
authService.AuthenticateAsync(TextBoxUsername.Text, TextBoxPassword.Text);
}
As you can see in the previous code, we need to create an eventhandler for the AuthenticateCompleted event.
void authService_AuthenticateCompleted(object sender, SilverlightAuthentication.AuthenticationService.AuthenticateCompletedEventArgs e)
{
if (e.Result)
{
LoginForm.Visibility = Visibility.Collapsed;
ResultForm.Visibility = Visibility.Visible;
TextBlockResult.Text = "";
}
else
{
TextBlockResult.Text = "Unable to log you in. Invalid username or password.";
}
}
In this piece of code we hide the LoginForm once the authentication is succeeded. If authentication fails, an errormessage is displayed in the TextBlockResult control.
When you press F5 to run the application, you should be able to login using the credentials we added in the Web.Config:
username: myUser
password: secret
If you use an incorrect username, the errormessage should appear in the TextBlockResult.
You can also try to use the "Get Xml File" button which should only work if you're logged in.
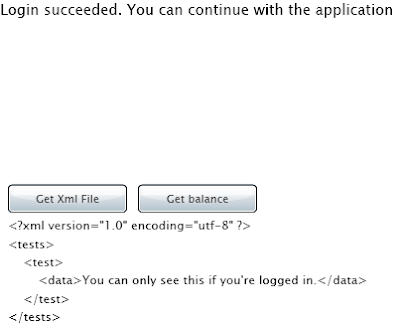
2.5 Create a secure WCF Webservice
For the retrieval of your balance (don't worry, it's not your real balance) we are going to create another Silverlight-enabled WCF service. Let's call it "BalanceService". Next, we'll create the method "GetMyBalance" in there which will return a decimal and a boolean value.
Again, replace the default generated method with the following code:
[OperationContract]
public bool GetMyBalance(out decimal Balance)
{
if (HttpContext.Current.User.Identity.IsAuthenticated)
{
Balance = 1000.00M;
return true;
}
else
{
Balance = decimal.MinValue;
return false;
}
}
As you can see, we use the User.Identity object, which was filled using the SetAuthCookie method we called earlier on in this tutorial, to check if the user calling the method is authenticated. If the user is authenticated, the webservice will return true and will fill the Balance output paramater with the correct value, else it will return false and the decimal.MinValue in the Balance parameter.
Next well add the functionality to call the WCF Service in the Silverlight application.
2.6 Add functionality in Silverlight for the BalanceService
First we need to add the Service Reference to the project again. Since we've done this before, I'll just sum it up:
- Right-click the Service References folder
- Click on Add Service Reference
- Click on Discover
- Select the service "BalanceService"
- Change the namespace to "BalanceService"
- Click OK
Once we're done with that it's time to do some coding.
Add the following line to the Page() constructor, just before the closing curly brace:
ButtonGetMyBalance.Click += new RoutedEventHandler(ButtonGetMyBalance_Click);
Next, create the event handler for the Click event:
void ButtonGetMyBalance_Click(object sender, RoutedEventArgs e)
{
BalanceService.BalanceServiceClient balanceService = new SilverlightAuthentication.BalanceService.BalanceServiceClient();
balanceService.GetMyBalanceCompleted += new EventHandler(balanceService_GetMyBalanceCompleted);
balanceService.GetMyBalanceAsync();
}
What we see here is that it is not necessary to add the out parameter of our method to the webservice call. In the next step we'll see what happens with that.
All we need to do now is to create the handler for the GetMyBalanceCompleted event:
private void balanceService_GetMyBalanceCompleted(object sender, SilverlightAuthentication.BalanceService.GetMyBalanceCompletedEventArgs e)
{
if (e.Result == true)
{
TextBlockResult.Text = string.Format("Your balance: {0}", e.Balance.ToString());
}
else
{
TextBlockResult.Text = "Please login first";
}
}
What we see now is that our out parameter is included in the GetMyBalanceCompletedEventArgs. This way we can use multiple parameters without creating a class for this.
If you press F5 now, and try the button without logging in, you'll see the "Please login first" text in the resultbox.
After you're logged in and press the button the Balance will appear in the resultbox.
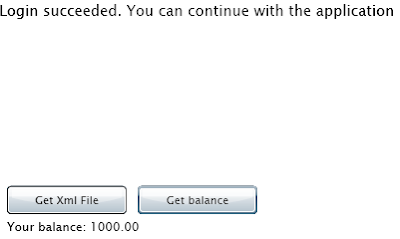
That's it for now. I hope this helps to get you started using this type of authentication.
Click here to download the source